Distributed Systems Lab
In this assignment, I implemented an electronic mail service. The core features are:
- a message transfer protocol (DMTP)
- a message access protocol (DMAP)
- transfer servers (responsible for forwarding messages to mailbox servers)
- mailbox servers (responsible for storing messages and making them available to users)
- monitoring server (receives usage statistics from transfer servers via UDP)
- a decentralized naming service (for managing addresses and locations of mailbox servers)
- opportunistic encryption for enabling secure DMAP channels
- allowing users to verify the integrity of messages
- a message client (making it easy for users to use the electronic mail service)
Technologies used:
- Java Cryptography API (JCA)
- Multithreading
- Java Remote Method Invocation (RMI)
- TCP and UDP sockets
- Java Dynamic Proxies
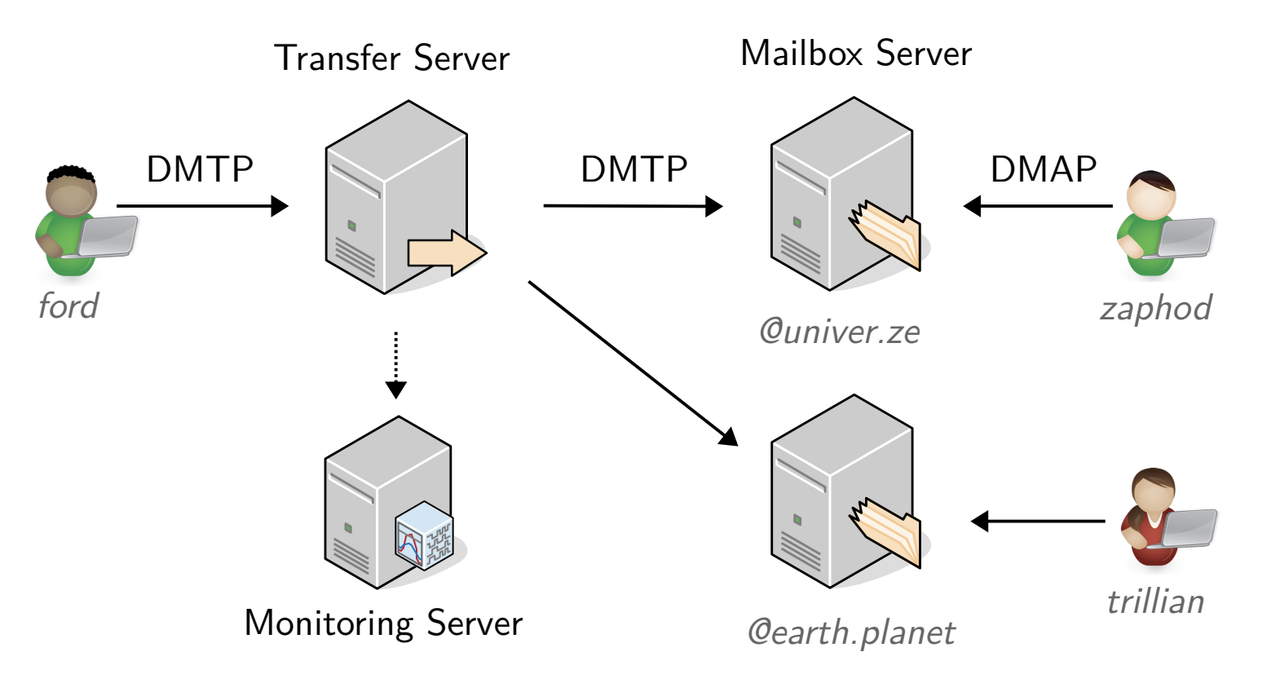
DMTP
The DSLab Message Transfer Protocol (DMTP) is a plaintext application-layer protocol that specifies a
way to exchange messages in a set of instructions:
- begin indicates the start of a message
- to address@foo.com,another@bar.com sets recipient(s)
- from me@univer.ze sets the sender
- subject How are you sets the subject
- data Want about eat lunch tonight at 6? sets the content of the message
- send finalizes the message and instructs the server to send it
- quit closes the socket connection
DMAP
DMAP is a plain text protocol that allows users
to access stored messages via a set of instructions:
- login trillian secret123 attempts to authenticate the user
- list lists all emails of the current user
- show 3 shows the message with id 3
- delete 3 deletes the message with the id 3
- logout ends the current session (but does not close the connection)
- quit closes the socket connection
Domain Name Service
Similar to DNS, our nameservers span a distributed namespace. The namespace
network is hierarchically divided into a collection of domains. There is a single top-level domain, hosted
by the root nameserver. Using nameservers, each domain can be divided into smaller subdomains (zones).
Servers can dynamically enter the network of name servers.
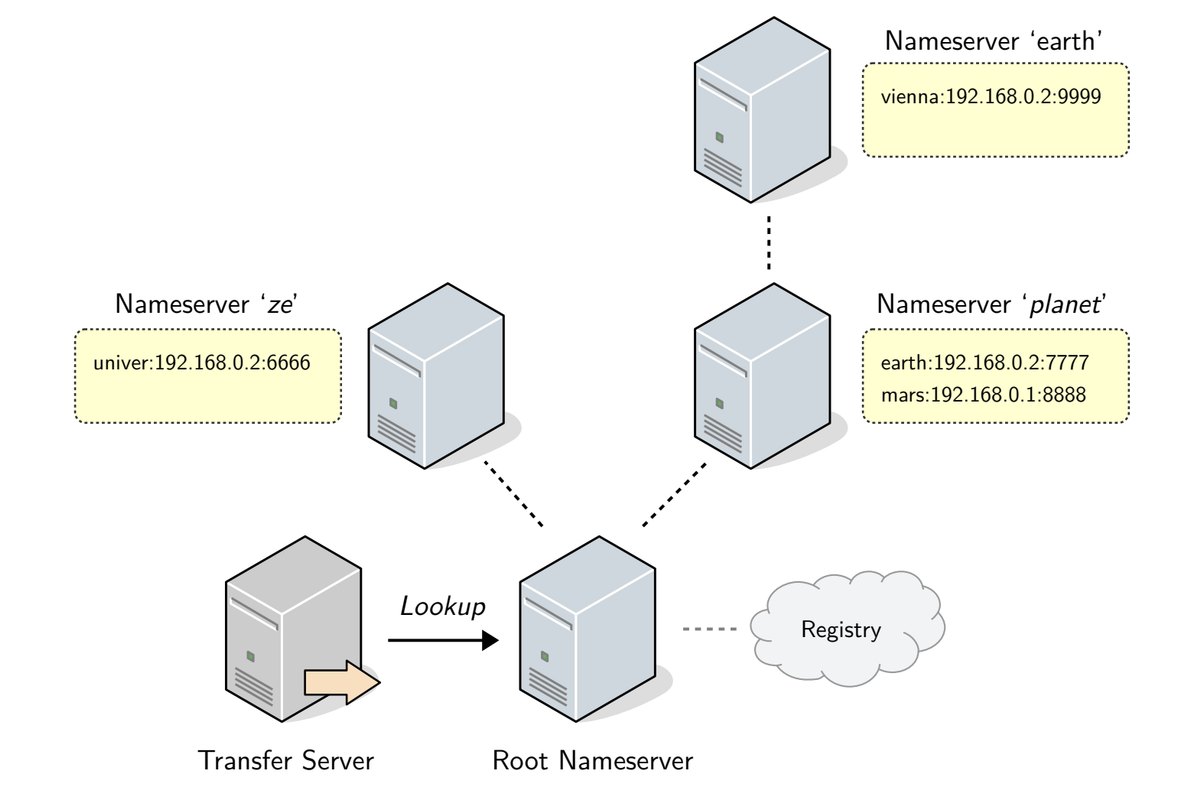
Message Client
The message client is a command-line application that provides the functionality
for
- communicating with DMAP/DMTP servers
- encryption and decryption
- message signing and verification
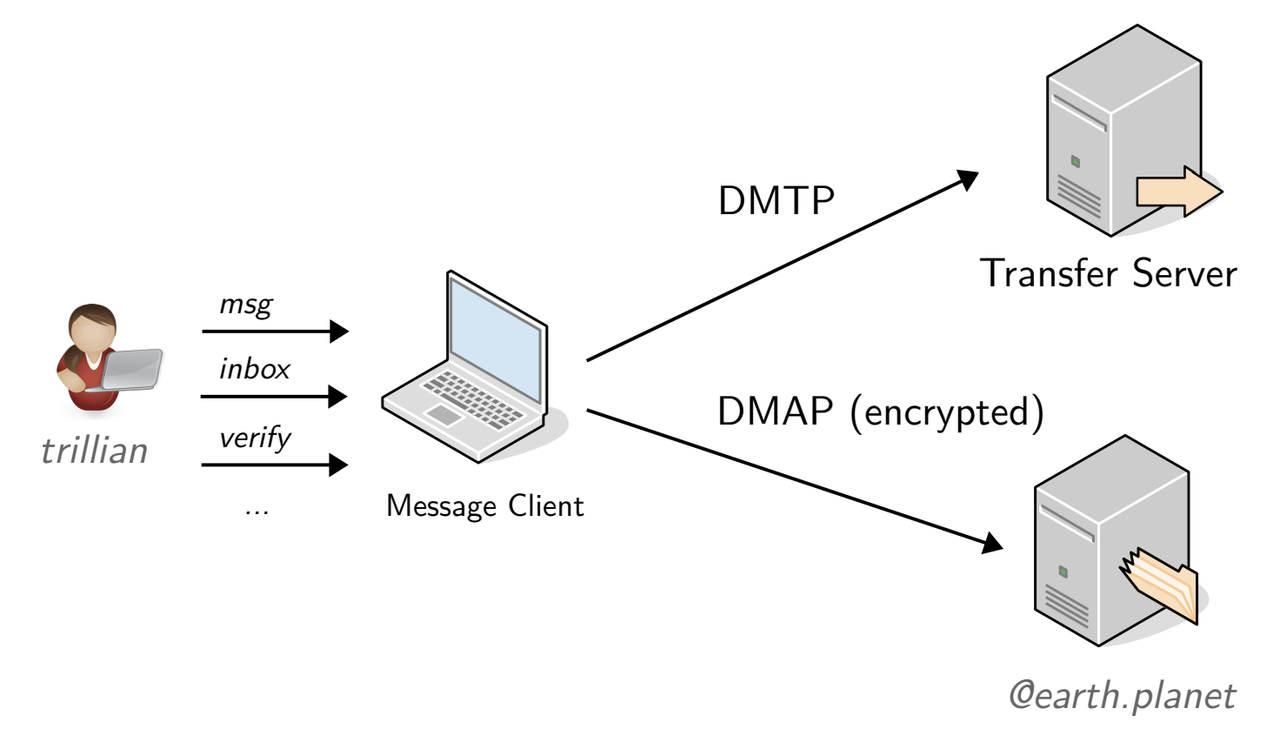
Using gradle
Compile & Test
Gradle is the build tool we are using. Here are some instructions:
Compile the project using the gradle wrapper:
./gradlew assemble
Compile and run the tests:
./gradlew build
Run the applications
The gradle config config contains several tasks that start application components for you.
You can list them with
./gradlew tasks --all
And search for 'Other tasks' starting with run-
. For example, to run the monitoring server, execute:
(the --console=plain
flag disables CLI features, like color output, that may break the console output when running a interactive application)
./gradlew --console=plain run-monitoring